This script retrieves email name and address from a selected message. However, when I reference it in the rules section of Mail preferences, I believe it exits because no messages are selected. I know I have it in the right place because other scripts in that folder are picked up by Mail. What am I doing wrong? I suspect I need something different than the select cmd.
tell application "Mail"
set selectedMessages to selection
if selectedMessages = {} then return
repeat with aMessage in selectedMessages
set senderName to extract name from sender of aMessage
set senderAddress to extract address from sender of aMessage
(*
display dialog " name: " & senderName & return & "address: " & senderAddress
*)
end repeat
end tell
display dialog "Blocked email(s) moved to 'Trash/On My Mac'.
" & "Name: " & senderName & return & "Address: " & senderAddress with title "Email Information"
What you want to do is use this script to work on the message that triggered the rule in Mail, is that correct?
I believe you need to include a handler to receive the command sent by mail.
Something like this:
using terms from application "Mail"
on perform mail action with messages theMessages
--Do your stuff here
end
end
But, the stuff your script does doesn’t necessarily have to do anything with theMessages. I just think when a rule triggers a script it needs a handler to receive the command. So, if you want it work on the message that triggered the command that would work. If you want it to actually work on selected messages, just ignore the theMessages variable.
@ estockly,
I did not really understand your answer. I am thinking I need to start from scratch. What I am trying to do is retrieve and display dialog the name and email address of the sender of the message that was triggered by the rule.
When I pasted my code where you said, and had that run, I believe no message data was given to the script.
@ estockly,
I added this to the library and triggered the rule. I did not get the test display dialog so I think the handler is not starting to run.
using terms from application "Mail"
on perform mail action with messages aMessage
tell application "Mail"
display dialog "aMessage is " & aMessage
if aMessage = {} then return
set senderName to extract name from sender of aMessage
set senderAddress to extract address from sender of aMessage
display dialog "Blocked email(s) moved to 'Trash/On My Mac'.
" & "Name: " & senderName & return & "Address: " & senderAddress with title "Email Information" with icon file (POSIX file "/Users/dracon/Documents/Misc Graphics/Icons/Icon Fix Mojave/Mail News Stocks/Mail Icon.png")
end tell
end perform mail action with messages
end using terms from
@estockly,
I modified it and got the display dialog which showed aMessage was blank. Please advise.
using terms from application "Mail"
set aMessage to {}
perform mail action with messages aMessage
on perform mail action with messages aMessage
tell application "Mail"
display dialog "aMessage is " & aMessage
if aMessage = {} then return
set senderName to extract name from sender of aMessage
set senderAddress to extract address from sender of aMessage
display dialog "Blocked email(s) moved to 'Trash/On My Mac'.
" & "Name: " & senderName & return & "Address: " & senderAddress with title "Email Information" with icon file (POSIX file "/Users/dracon/Documents/Misc Graphics/Icons/Icon Fix Mojave/Mail News Stocks/Mail Icon.png")
end tell
end perform mail action with messages
end using terms from
Here is how it works:
using terms from application "Mail"
on perform mail action with messages theMessages
set iconFile to "/Users/123/Downloads/TEST files/Moves.icns" as POSIX file
tell application "Mail"
repeat with aMessage in theMessages
display dialog "aMessage is " & (subject of aMessage)
set senderName to extract name from sender of aMessage
set senderAddress to extract address from sender of aMessage
display dialog "Blocked email(s) moved to 'Trash/On My Mac'." & return & ¬
"Name: " & senderName & return & "Address: " & ¬
senderAddress with title "Email Information" with icon iconFile
end repeat
end tell
end perform mail action with messages
end using terms from
I ran your script as a test triggered by a rule (see screenshot). When I removed the move message action at the top, the script functioned and the display dialogs executed. However, the repeat loop never stopped so that I had to respond to the dialog with Cancel - not OK. Maybe I could live with that but WITH the move action at the top, the script never displayed any dialogs as if it never received message information. There does not seem to be any way to change the order of the actions. It’s like the script can’t work if you move the message first and moving a message cannot happen last.
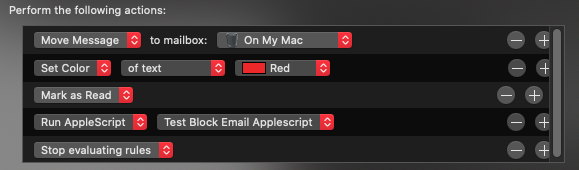
@KniazidisR ,
I tried using the script to also delete the message and it seems to work. So I can remove the move to trash action so that the script will actually work. Now when the rule detects a blocked email, the script displays the information and then deletes it (moves it to trash).
using terms from application "Mail"
on perform mail action with messages theMessages
set iconFile to "/Users/dracon/Documents/Misc Graphics/Icons/Icon Fix Mojave/Mail News Stocks/Mail Icon.png" as POSIX file
tell application "Mail"
set aMessage to (item 1 of theMessages)
(*
display dialog "aMessage is " & (subject of aMessage)
*)
set Sub to (subject of aMessage)
set senderName to extract name from sender of aMessage
set senderAddress to extract address from sender of aMessage
display dialog Sub & "
Blocked eMail will be deleted" & return & ¬
"From: " & senderName & return & "Address: " & ¬
senderAddress with title "Email Information" with icon iconFile
delete aMessage
(*
set theAccount to account of mailbox of aMessage
set mailbox of aMessage to mailbox "Trash" of theAccount
*)
end tell
end perform mail action with messages
end using terms from